Core methods#
laygo2.object.grid module contains classes that implement abstract coordinate systems that are interacting with technology-specific physical coordinate systems.
laygo2 implements the layout designs based on the abstract coordinate system.
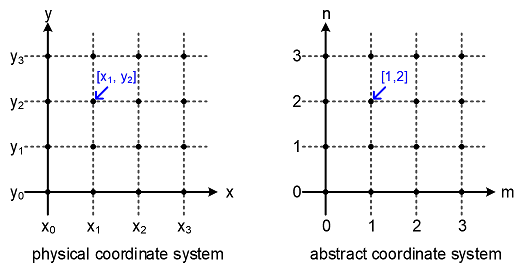
Check the following links for the details of component methods and classes.
- laygo2.object.grid.copy(obj)[source]#
Make a copy of the input grid object.
- Parameters:
obj (laygo2.object.grid.Grid) – The input grid object to be copied.
- Returns:
laygo2.object.grid.Grid or derived
- Return type:
the copied grid object.
Example
>>> import laygo2 >>> from laygo2.object.grid import OneDimGrid, Grid >>> g1_x = OneDimGrid(name='xgrid', scope=[0, 100], elements=[0, 10, 20, 40, 50 ]) >>> g1_y = OneDimGrid(name='ygrid', scope=[0, 100], elements=[10, 20, 40, 50, 60 ]) >>> g2 = Grid(name="test", vgrid = g1_x, hgrid = g1_y ) >>> g2_copy = laygo2.object.grid.copy(g2) >>> print(g2) <laygo2.object.grid.core.Grid object at 0x000002002EBA67A0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])], >>> print(g2_copy) <laygo2.object.grid.core.Grid object at 0x0000020040C35240> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])],
- laygo2.object.grid.vflip(obj)[source]#
Make a vertically-flipped copy of the input grid object.
- Parameters:
obj (laygo2.object.grid.Grid) – The input grid object to be copied and flipped.
- Returns:
laygo2.object.grid.Grid or derived
- Return type:
the generated grid object.
Example
>>> import laygo2 >>> from laygo2.object.grid import OneDimGrid, Grid >>> g1_x = OneDimGrid(name='xgrid', scope=[0, 100], elements=[0, 10, 20, 40, 50 ]) >>> g1_y = OneDimGrid(name='ygrid', scope=[0, 100], elements=[10, 20, 40, 50, 60 ]) >>> g2 = Grid(name="test", vgrid = g1_x, hgrid = g1_y ) >>> g2_copy = laygo2.object.grid.vflip(g2) >>> print(g2) <laygo2.object.grid.core.Grid object at 0x000001EE82660BE0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])], >>> print(g2_copy) <laygo2.object.grid.core.Grid object at 0x000001EE947152D0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([40, 50, 60, 80, 90])],
- laygo2.object.grid.hflip(obj)[source]#
Make a horizontally-flipped copy of the input grid object.
- Parameters:
obj (laygo2.object.grid.Grid) – The input grid object to be copied and flipped.
- Returns:
laygo2.object.grid.Grid
- Return type:
the generated grid object.
Example
>>> import laygo2 >>> from laygo2.object.grid import OneDimGrid, Grid >>> g1_x = OneDimGrid(name='xgrid', scope=[0, 100], elements=[0, 10, 20, 40, 50 ]) >>> g1_y = OneDimGrid(name='ygrid', scope=[0, 100], elements=[10, 20, 40, 50, 60 ]) >>> g2 = Grid(name="test", vgrid = g1_x, hgrid = g1_y ) >>> g2_copy = laygo2.object.grid.hflip(g2) >>> print(g2) <laygo2.object.grid.core.Grid object at 0x000001ACECC30BE0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])], >>> print(g2_copy) <laygo2.object.grid.core.Grid object at 0x000001ACFED15300> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 50, 60, 80, 90, 100]), array([10, 20, 40, 50, 60])],
- laygo2.object.grid.hstack(obj)[source]#
Stack grid(s) in horizontal direction.
- Parameters:
obj (list of laygo2.object.grid.Grid) – The list containing grid objects to be stacked.
- Returns:
laygo2.object.grid.Grid
- Return type:
the generated grid object.
Example
>>> import laygo2 >>> from laygo2.object.grid import OneDimGrid, Grid >>> g1_x = OneDimGrid(name='xgrid', scope=[0, 100], elements=[0, 10, 20, 40, 50 ]) >>> g1_y = OneDimGrid(name='ygrid', scope=[0, 100], elements=[10, 20, 40, 50, 60 ]) >>> g2 = Grid(name="test", vgrid = g1_x, hgrid = g1_y ) >>> g2_copy = laygo2.object.grid.copy(g2) >>> g2_stack = laygo2.object.grid.hstack([g2, g2_copy]) >>> print(g2) <laygo2.object.grid.core.Grid object at 0x000001799FAA0BE0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])], >>> print(g2_stack) <laygo2.object.grid.core.Grid object at 0x0000015BD8C85570> name: test, class: Grid, scope: [[0, 0], [200, 100]], elements: [array([ 0, 10, 20, 40, 50, 100, 110, 120, 140, 150]), array([10, 20, 40, 50, 60])],
- laygo2.object.grid.vstack(obj)[source]#
Stack grid(s) in vertical direction.
- Parameters:
obj (list of laygo2.object.grid.Grid) – The list containing grid objects to be stacked.
- Returns:
laygo2.object.grid.Grid
- Return type:
the generated grid object.
Example
>>> import laygo2 >>> from laygo2.object.grid import OneDimGrid, Grid >>> g1_x = OneDimGrid(name='xgrid', scope=[0, 100], elements=[0, 10, 20, 40, 50 ]) >>> g1_y = OneDimGrid(name='ygrid', scope=[0, 100], elements=[10, 20, 40, 50, 60 ]) >>> g2 = Grid(name="test", vgrid = g1_x, hgrid = g1_y ) >>> g2_copy = laygo2.object.grid.copy(g2) >>> g2_stack = laygo2.object.grid.vstack([g2, g2_copy]) >>> print(g2) <laygo2.object.grid.core.Grid object at 0x000001799FAA0BE0> name: test, class: Grid, scope: [[0, 0], [100, 100]], elements: [array([ 0, 10, 20, 40, 50]), array([10, 20, 40, 50, 60])], >>> print(g2_stack) <laygo2.object.grid.core.Grid object at 0x00000179B1B05870> name: test, class: Grid, scope: [[0, 0], [100, 200]], elements: [array([ 0, 10, 20, 40, 50]), array([ 10, 20, 40, 50, 60, 110, 120, 140, 150, 160])],